三角関数
三角関数は、直角三角形で1つの角が決まると、\(\displaystyle \sin \)、\(\displaystyle \cos \)、\(\displaystyle \tan \)によって、斜辺、底辺、高さの比が決まる関数です。
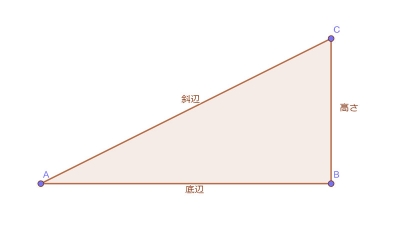
\(\displaystyle \angle A \)を\(\displaystyle \angle \theta \)とすると次のように表すことができる。
正弦 : \(\displaystyle \sin \theta = \frac{高さ}{斜辺} \)
余弦 : \(\displaystyle \cos \theta = \frac{底辺}{斜辺} \)
正接 : \(\displaystyle \tan \theta = \frac{高さ}{底辺} \)
また、sin、cos、tanのそれぞれの値は次のように求められる。
正弦 : \(\displaystyle \sin z = \sum_{n=0}^\infty \frac{(-1)^n}{(2n + 1)!} z^{2n + 1} \)
余弦 : \(\displaystyle \cos z = \sum_{n=0}^\infty \frac{(-1)^n}{(2n)!} z^{2n} \)
正接 : \(\displaystyle \tan \theta = \frac{\sin z}{\cos z} \)
Pythonでsinとcosを描画する
3つの山がある\(\displaystyle \sin \)と\(\displaystyle \cos \)を描画してみる。
import numpy as np
from matplotlib import pylab as plt
# 3つの山を作る
p_range = 3 * np.pi
p = np.linspace(-p_range, p_range, 100)
# sinを描画する
plt.plot(np.sin(p))
# cosを描画する
plt.plot(np.cos(p))
plt.title('sin, cos')
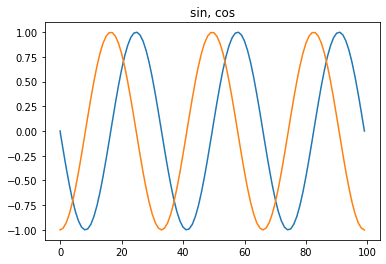
オレンジの線がsinの線で、直角三角形の高さを表しており、水色の線がcosの線で、直角三角形の底辺を表している。
Pythonでsinとcosとtanを求める
次はsin、cos、tanの値を求めてみる。
正弦 : \(\displaystyle \sin z = \sum_{n=0}^\infty \frac{(-1)^n}{(2n + 1)!} z^{2n + 1} \)
余弦 : \(\displaystyle \cos z = \sum_{n=0}^\infty \frac{(-1)^n}{(2n)!} z^{2n} \)
正接 : \(\displaystyle \tan \theta = \frac{\sin z}{\cos z} \)
import math
import numpy as np
import pandas as pd
def sin_func(z, n_max=100):
"""
sinを求める関数
param z : 角度
param n_max : 総和のn
return s : sinの値
"""
s = 0
for n in range(n_max):
m = 2 * n + 1
s += (((-1) ** n) / math.factorial(m)) * (z ** m)
return s
def cos_func(z, n_max=100):
"""
cosを求める関数
param z : 角度
param n_max : 総和のn
return c : cosの値
"""
c = 0
for n in range(n_max):
m = 2 * n
c += (((-1) ** n) / math.factorial(m)) * (z ** m)
return c
# tanを求める関数
def tan_func(z, n_max=100):
"""
sinを求める関数
param z : 角度
param n_max : 総和のn
return t : tanの値
"""
t = 0
t = sin_func(z, n_max) / cos_func(z, n_max)
return t
# ラジアンの配列
rad = np.arange(0, 5, 0.5)
sin_data = pd.DataFrame([[sin_func(r)] for r in rad], index=rad, columns=['sinの値'])
cos_data = pd.DataFrame([[cos_func(r)] for r in rad], index=rad, columns=['cosの値'])
tan_data = pd.DataFrame([[tan_func(r)] for r in rad], index=rad, columns=['tanの値'])
data = sin_data.join(cos_data).join(tan_data)
print(data)
sinの値 cosの値 tanの値
0.0 0.000000 1.000000 0.000000
0.5 0.479426 0.877583 0.546302
1.0 0.841471 0.540302 1.557408
1.5 0.997495 0.070737 14.101420
2.0 0.909297 -0.416147 -2.185040
2.5 0.598472 -0.801144 -0.747022
3.0 0.141120 -0.989992 -0.142547
3.5 -0.350783 -0.936457 0.374586
4.0 -0.756802 -0.653644 1.157821
4.5 -0.977530 -0.210796 4.637332
numpyでは、sin、cos、tanをそれぞれnp.sin、np.cos、np.tanで求められる。
import numpy as np
import pandas as pd
rad = np.arange(0, 5, 0.5)
sin_data = pd.DataFrame([[np.sin(r)] for r in rad], index=rad, columns=['sinの値'])
cos_data = pd.DataFrame([[np.cos(r)] for r in rad], index=rad, columns=['cosの値'])
tan_data = pd.DataFrame([[np.tan(r)] for r in rad], index=rad, columns=['tanの値'])
data = sin_data.join(cos_data).join(tan_data)
print(data)
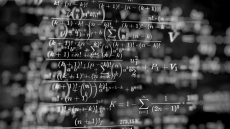
記事を読んでいただきありがとうございました。